KOTLIN
Certainly! Here’s a brief overview of basic Kotlin concepts:
- Variables and Constants: In Kotlin, you declare variables using
var
and constants (immutable variables) usingval
. For example:kotlinCopy codevar x = 10 val y = 20
- Data Types: Kotlin provides various data types such as Int, Long, Float, Double, Boolean, Char, etc. The type can be inferred by the compiler, but you can also explicitly specify it.kotlinCopy code
val name: String = "John" val age: Int = 30
- Functions: Functions are declared using the
fun
keyword. Functions can have parameters and return types.kotlinCopy codefun add(x: Int, y: Int): Int { return x + y }
- Classes and Objects: You define classes using the
class
keyword. Instances of classes are created using thenew
keyword.kotlinCopy codeclass Person(val name: String, val age: Int) val person = Person("Alice", 25)
- Null Safety: Kotlin has a strong emphasis on null safety. Variables cannot hold null values by default, but you can declare nullable types by appending
?
to the type.kotlinCopy codevar nullableString: String? = null
- Control Flow: Kotlin supports traditional control flow structures like
if
,when
,for
, andwhile
.when
is similar to the switch statement in other languages.kotlinCopy codeval score = 85 when { score >= 90 -> println("A") score >= 80 -> println("B") else -> println("C") }
- Collections: Kotlin provides a rich set of collections such as List, Set, and Map. They can be created using constructors or using convenience methods.kotlinCopy code
val numbersList = listOf(1, 2, 3, 4, 5) val numbersSet = setOf(1, 2, 3, 4, 5) val map = mapOf("key1" to 1, "key2" to 2)
- Extension Functions: Kotlin allows you to add new functionality to existing classes without modifying their code by using extension functions.kotlinCopy code
fun Int.isEven(): Boolean { return this % 2 == 0 } println(4.isEven()) // true
- Lambda Expressions: Kotlin supports lambda expressions which are often used for functional programming constructs like
map
,filter
,reduce
, etc.kotlinCopy codeval numbers = listOf(1, 2, 3, 4, 5) val squaredNumbers = numbers.map { it * it }
This is just a brief overview of Kotlin’s basic concepts. There’s much more to explore, including coroutines, properties, delegation, and more.
1. Before you begin
Install Android Studio on your computer if you haven’t done so already. Check that your computer meets the system requirements required for running Android Studio (located at the bottom of the download page). If you need more detailed instructions on the setup process, refer to the Download and install Android Studio codelab.
In this codelab, you create your first Android app with a project template provided by Android Studio. You use Kotlin and Jetpack Compose to customize your app. Note that Android Studio gets updated and sometimes the UI changes so it is okay if your Android Studio looks a little different than the screenshots in this codelab.
Prerequisites
- Basic Kotlin knowledge
What you’ll need
- The latest version of Android Studio
What you’ll learn
- How to create an Android App with Android Studio
- How to run apps with the Preview tool in Android Studio
- How to update text with Kotlin
- How to update a User Interface (UI) with Jetpack Compose
- How to see a preview of your app with Preview in Jetpack Compose
What you’ll build
- An app that lets you customize your introduction!
Here’s what the app looks like when you complete this codelab (except it will be customized with your name!):
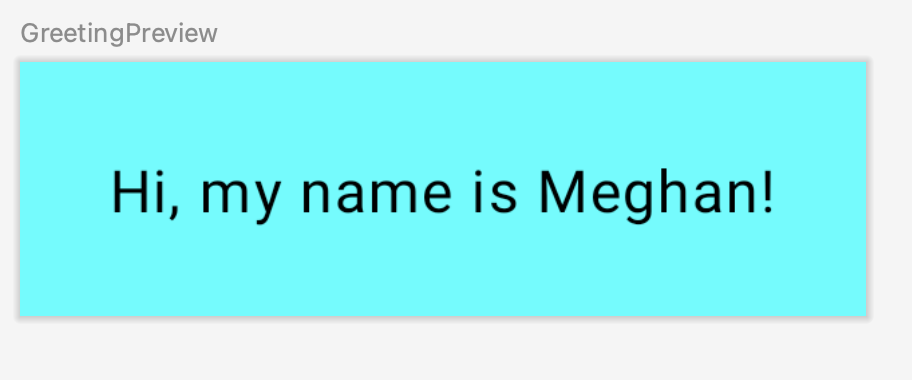
What you’ll need
- A computer with Android Studio installed.
2. Create a project using the template
In this codelab, you create an Android app with the Empty Activity project template provided by Android Studio.
To create a project in Android Studio:
- Double click the Android Studio icon to launch Android Studio.
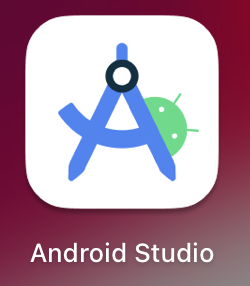
- In the Welcome to Android Studio dialog, click New Project.
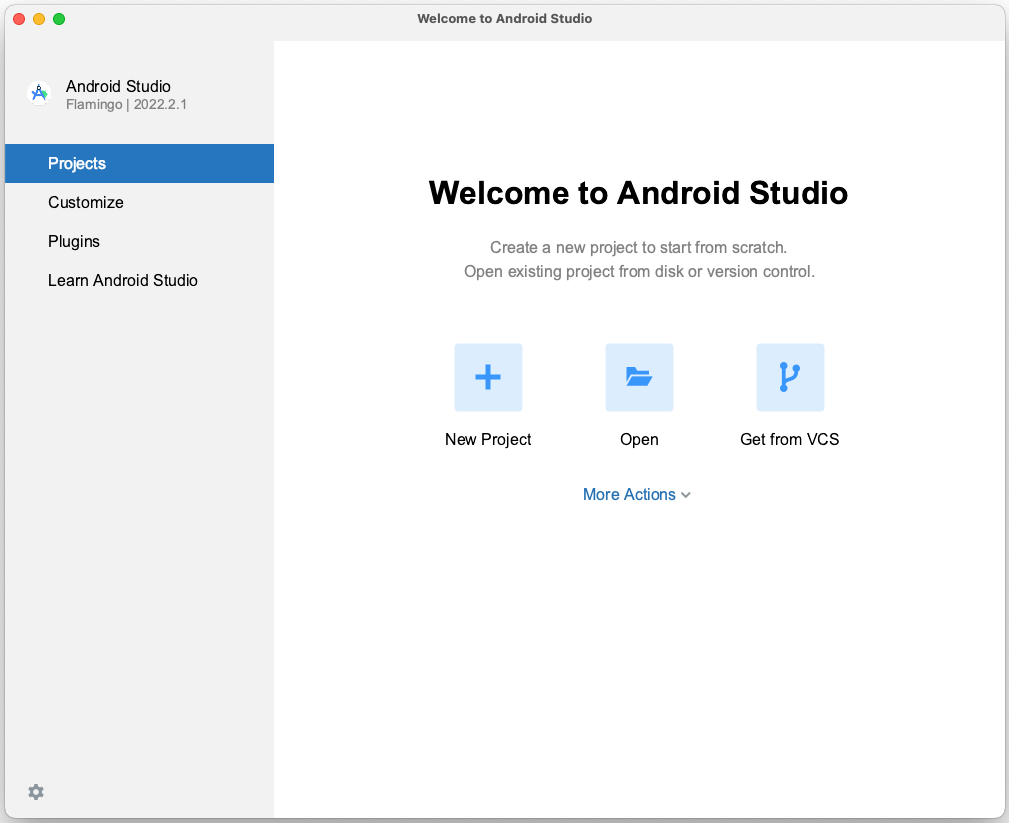
The New Project window opens with a list of templates provided by Android Studio.
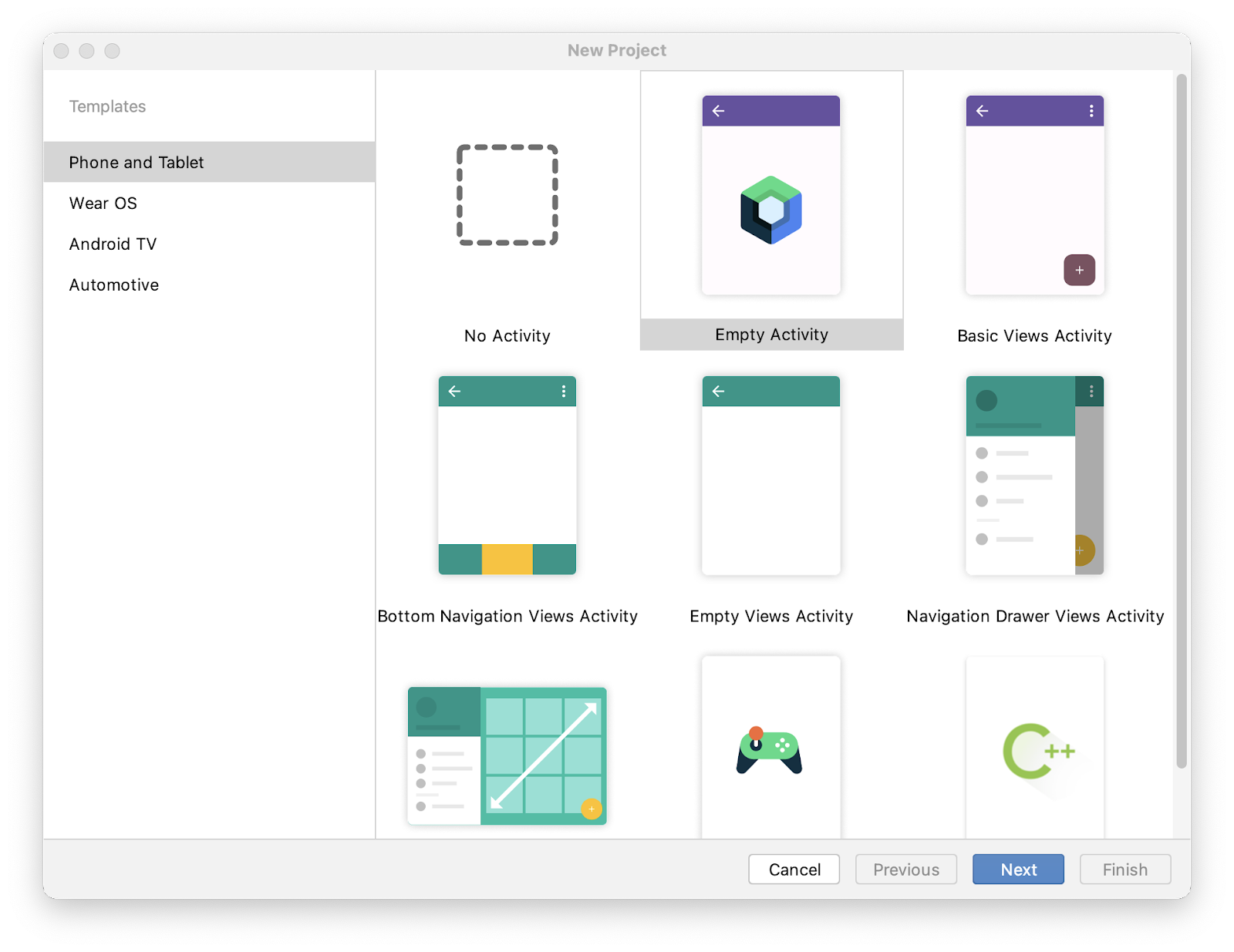
In Android Studio, a project template is an Android project that provides the blueprint for a certain type of app. Templates create the structure of the project and the files needed for Android Studio to build your project. The template that you choose provides starter code to get you going faster.
- Make sure the Phone and Tablet tab is selected.
- Click the Empty Activity template to select it as the template for your project. The Empty Activity template is the template to create a simple project that you can use to build a Compose app. It has a single screen and displays the text
"Hello
Android!"
. - Click Next. The New Project dialog opens. This has some fields to configure your project.
- Configure your project as follows:
The Name field is used to enter the name of your project, for this codelab type “Greeting Card”.
Leave the Package name field as is. This is how your files will be organized in the file structure. In this case, the package name will be com.example.greetingcard
.
Leave the Save location field as is. It contains the location where all the files related to your project are saved. Take a note of where that is on your computer so that you can find your files.
Select API 24: Android 7.0 (Nougat) from the menu in the Minimum SDK field. Minimum SDK indicates the minimum version of Android that your app can run on.
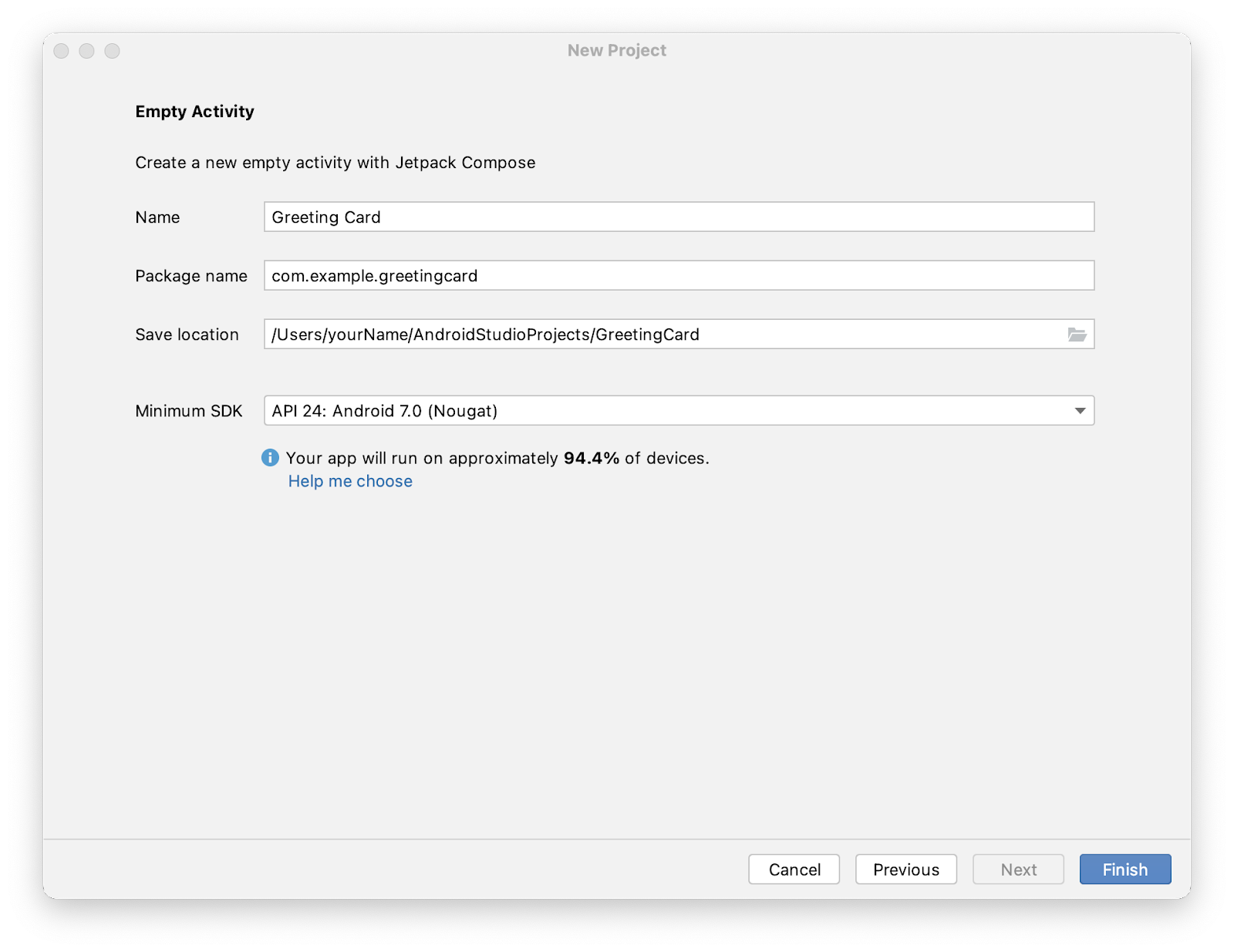
- Click Finish. This may take a while – this is a great time to get a cup of tea! While Android Studio is setting up, a progress bar and message indicates whether Android Studio is still setting up your project. It may look like this:

A message that looks similar to this informs you when the project set up is created.

- You may see a What’s New pane which contains updates on new features in Android Studio. Close it for now.
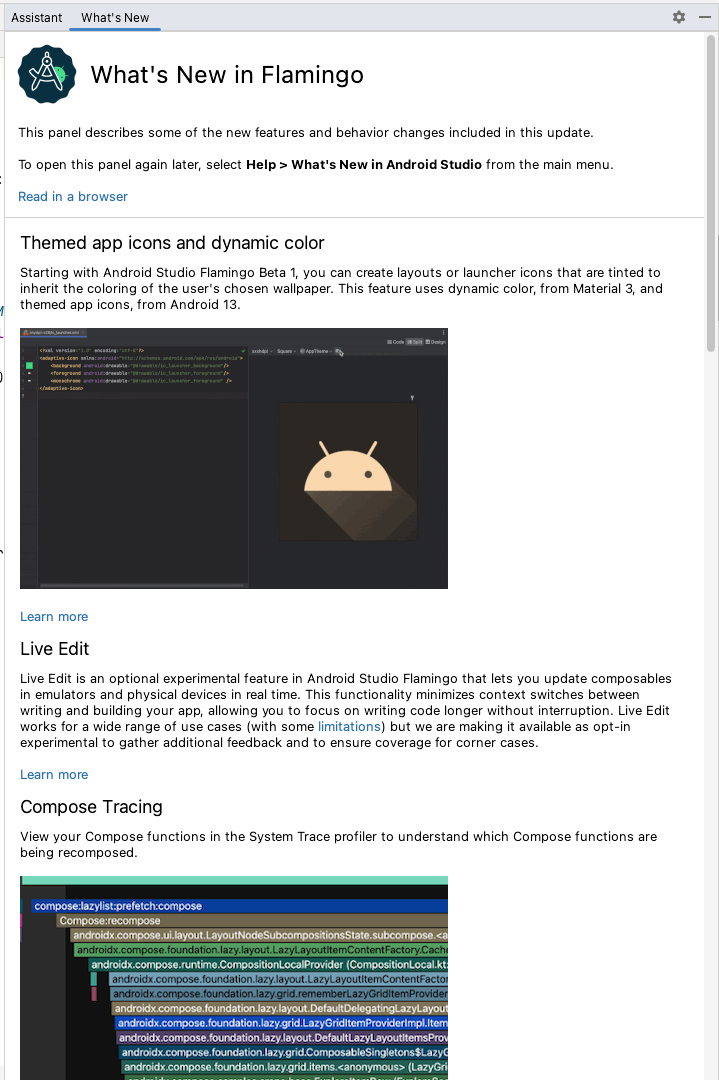
- Click Split on the top right of Android Studio, this allows you to view both code and design. You can also click Code to view code only or click Design to view design only.

After pressing Split you should see three areas:
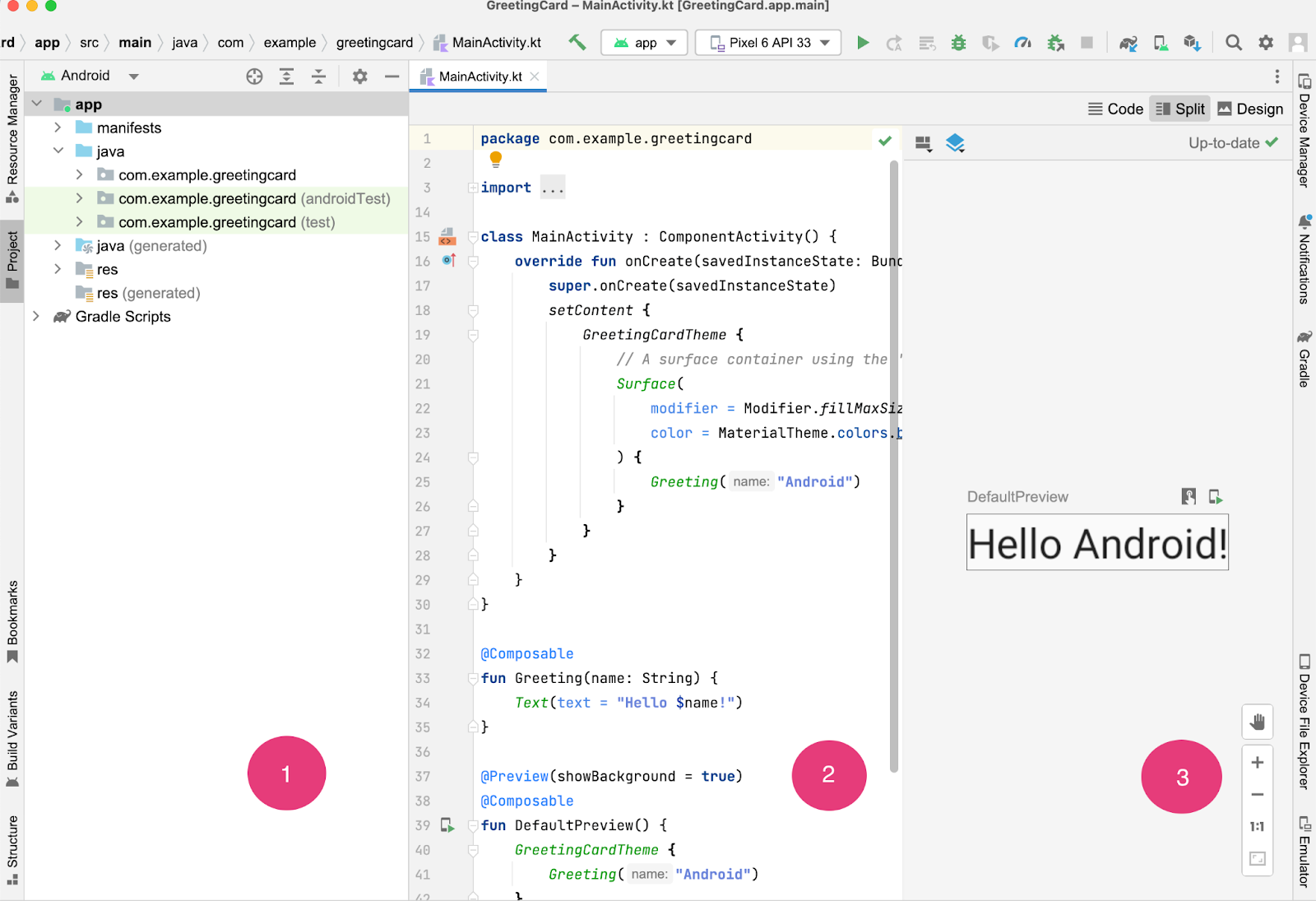
- The Project view (1) shows the files and folders of your project
- The Code view (2) is where you edit code
- The Design view (3) is where you preview what your app looks like
In the Design view, you will see a blank pane with this text:
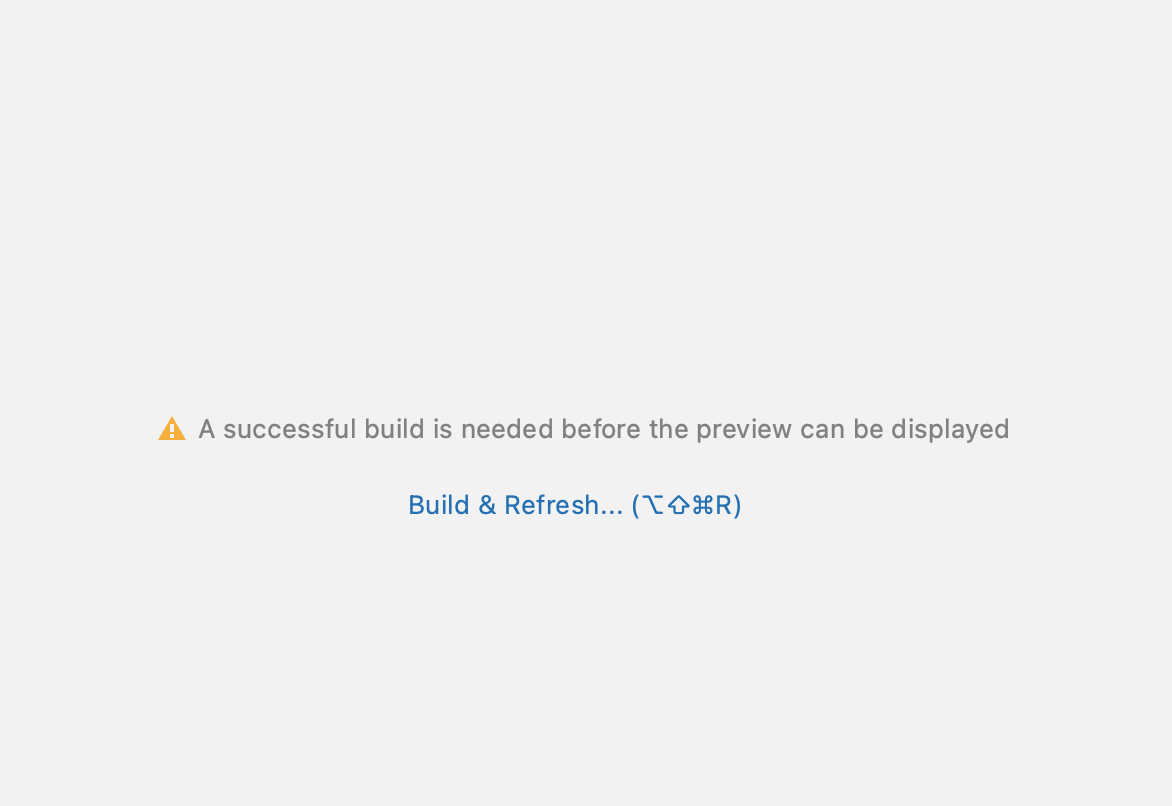
- Click Build & Refresh. It may take a while to build but when it is done the preview shows a text box that says “Hello Android!“. Empty Compose activity contains all the code necessary to create this app.
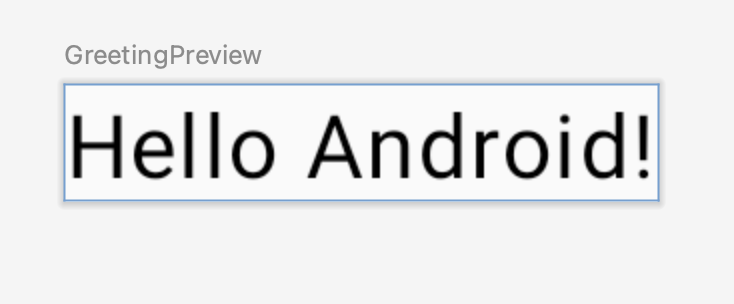
DownloadAndroid Studio editorAndroid Gradle PluginSDK toolsPreview
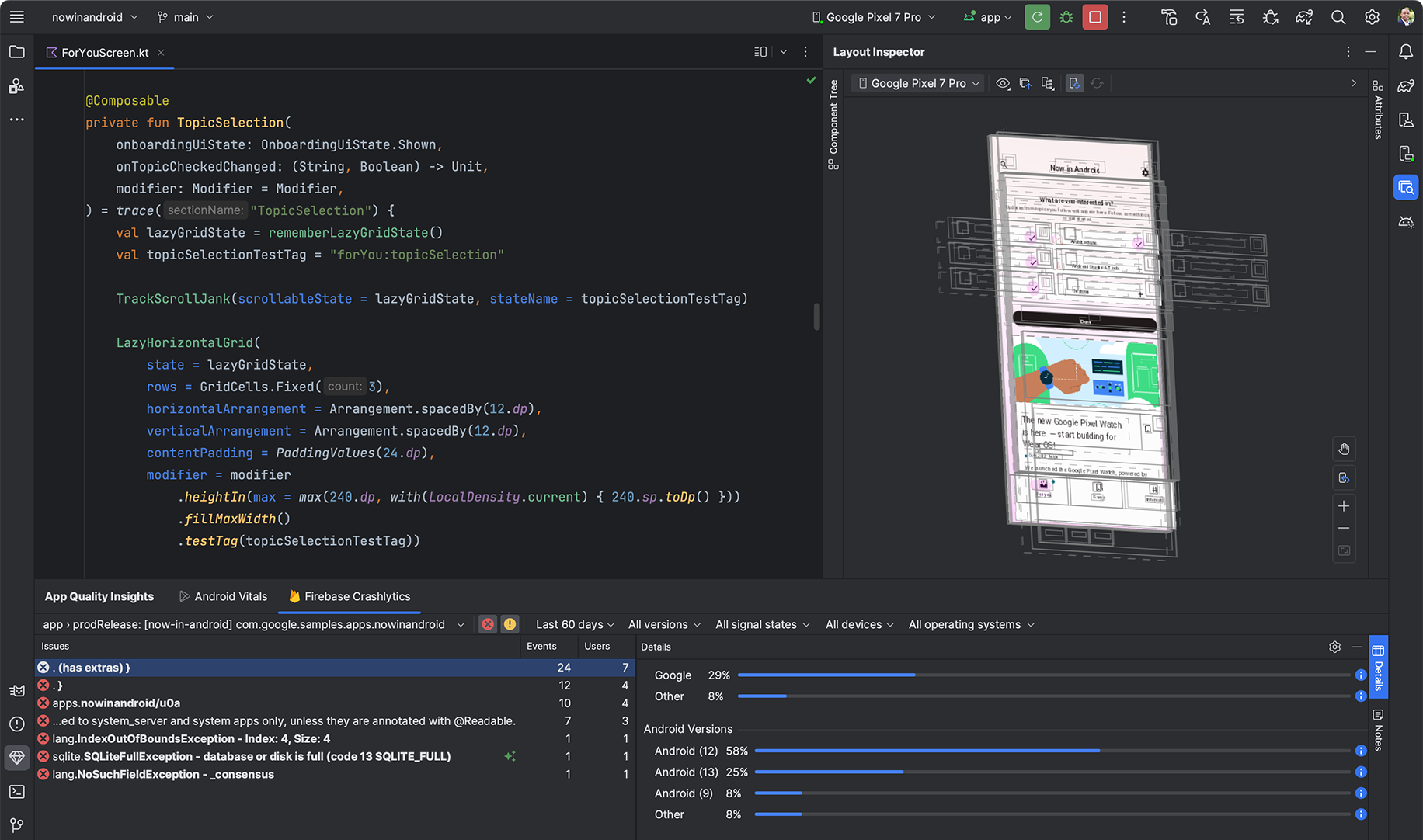
Android Studio
Get the official Integrated Development Environment (IDE) for Android app development.
Download Android Studio Iguana
New features
FEATURE
Themed app icon preview
Preview how your themed app icons respond to dynamic background wallpaper changes.
FEATURE
Try Android Studio Bot
Studio Bot is an AI assistant that helps you generate code, fix code, and answer questions about Android app development. Available in Android Studio Jellyfish canary.
Core features
DESIGN
Compose design tools
Create dynamic layouts with Jetpack Compose. Then preview your layouts on any screen size and inspect Compose animations using the built-in inspection tools.
DEVELOP
Intelligent code editor
Write better code, work faster, and be more productive with an intelligent code editor that provides code completion for Kotlin, Java, and C/C++ programing languages. Moreover, when editing Jetpack Compose you can see your code changes reflected immediately with Live Edit.
BUILD
Flexible build system
Powered by Gradle, Android Studio’s build system lets you to customize your build to generate multiple build variants for different Android devices from a single project. Then analyze the performance of your builds and understand where potential build issues exist in your project with the Build Analyzer.
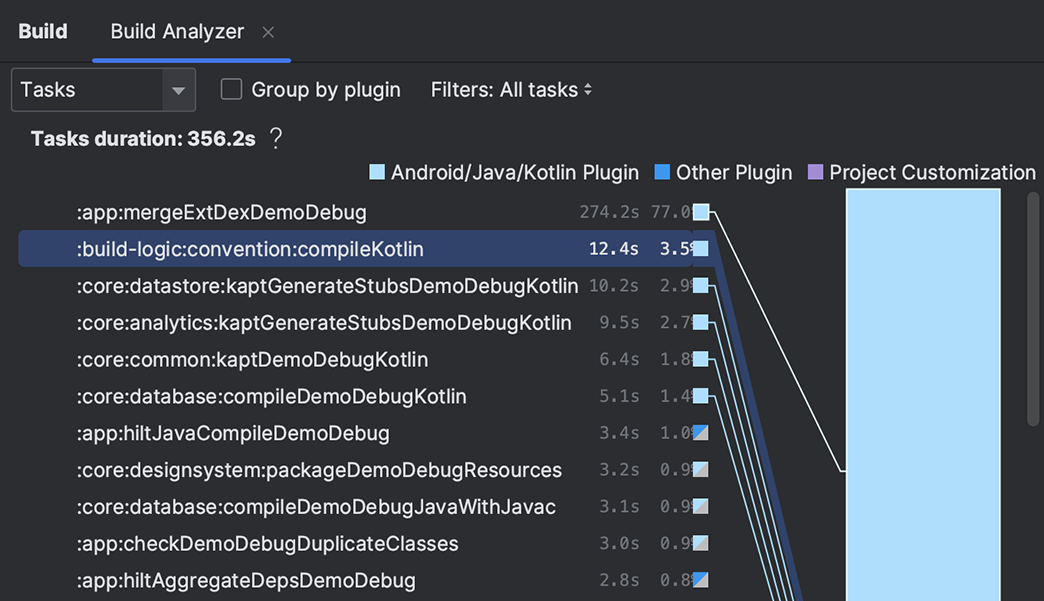
TEST
Easily emulate any device
The Android Emulator lets you to test your application on a variety of Android devices. Unlock the full potential of your apps by using responsive layouts that adapt to fit phones, tablets, foldables, Wear OS, TV and ChromeOS devices.
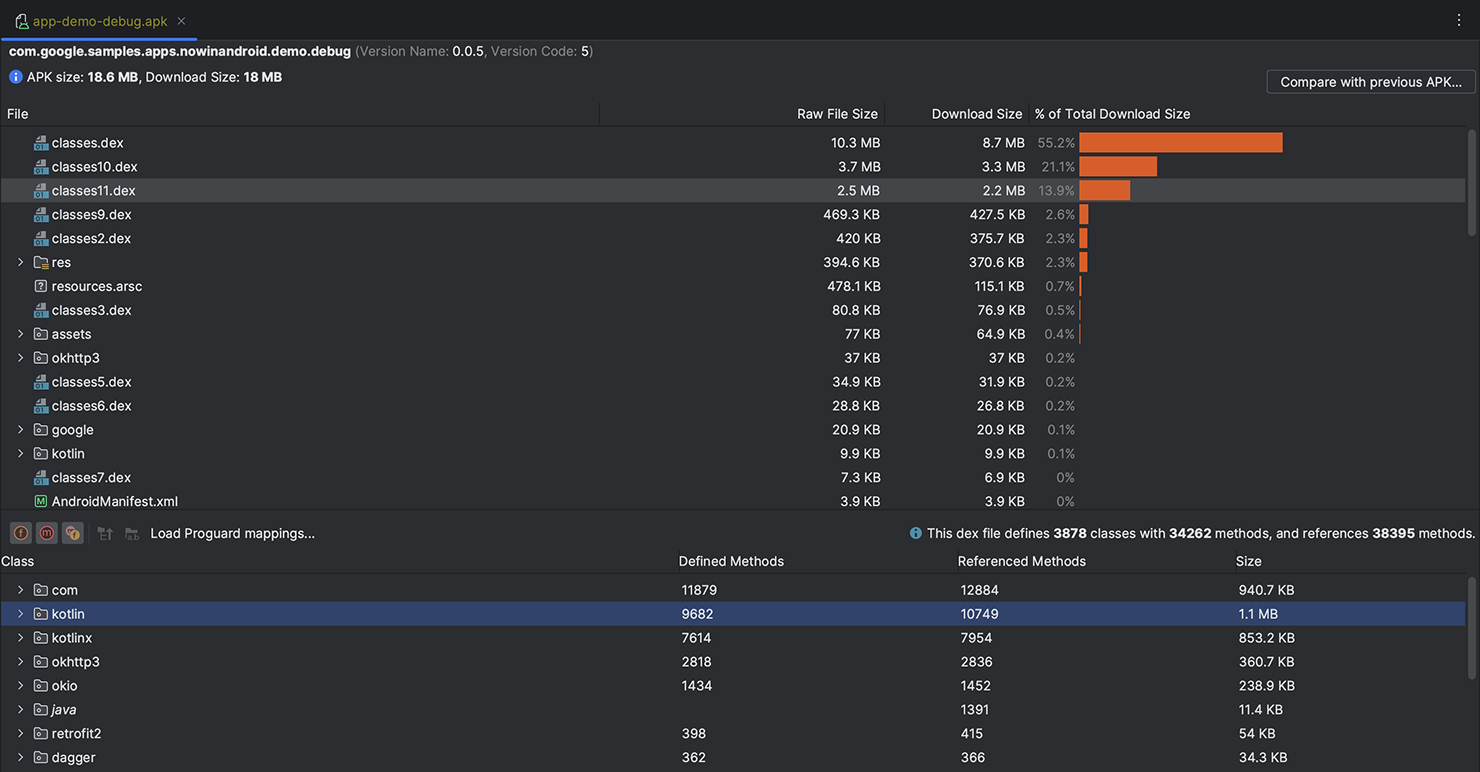
PUBLISH
Android App Bundle
Find opportunities to optimize your Android app size before publishing by inspecting the contents of your app APK file or Android App Bundle. Inspect the manifest file, resources, and DEX files. Compare two APKs or Android App Bundles to see how your app size changed between app versions.
Get started with Android Studio
Getting started
Learn how to set up your app development environment, configure Android Studio, and start writing an Android app.
GETTING STARTED
Install Android Studio
Android Studio can be installed on Microsoft® Windows®, MacOS®, Linux, and ChromeOS. Learn how to install it in a few simple clicks.
GETTING STARTED
Build your first app
Learn how to build a simple Android app in Android Studio by creating a “Hello, World!” project.
GETTING STARTED
Configure Android Studio
Learn to configure Android Studio according to system requirements, create preferred default settings, customize virtual machine options, and more.
GETTING STARTED
Training for beginners and pros
Whether you’re new to Android app development or just updating your skills, we offer training courses for a variety of levels and topics.
Android Studio downloads
Download the latest version of Android Studio. For more information, see the Android Studio release notes.
Platform | Android Studio package | Size | SHA-256 checksum |
---|---|---|---|
Windows (64-bit) | android-studio-2023.2.1.24-windows.exe Recommended | 1.1 GB | e2f70d192b2ed97d13901b63ae777672961a8b6ff1d86cae65fd26a5688d0d41 |
Windows (64-bit) | android-studio-2023.2.1.24-windows.zip No .exe installer | 1.2 GB | da1c548b6d57f1dd63737cb248cf6760eddbd79ab21e8b1e3c3b55123ab4ef57 |
Mac (64-bit) | android-studio-2023.2.1.24-mac.dmg | 1.2 GB | 1b7e1d5da8d88dadff7276d775372fa25e97a2bda2682fff7494f7ef46a93390 |
Mac (64-bit, ARM) | android-studio-2023.2.1.24-mac_arm.dmg | 1.2 GB | 985f39d146b6534f256694dec90a158a412a39b0da53f58bc135559703f7a830 |
Linux (64-bit) | android-studio-2023.2.1.24-linux.tar.gz | 1.2 GB | 0026427572849c9cbb0c94d6f9718ea08bc345dccfe3b372b54100a95fff99b5 |
ChromeOS | android-studio-2023.2.1.24-cros.deb | 944.2 MB | 4c0055429aa7ab10e7c2b870e8e3c03ca191c913d8f91474f61ea1b5d048e9f8 |
More downloads are available in the download archives. For Android Emulator downloads, see the Emulator download archives.
Command line tools only
Platform | SDK tools package | Size | SHA-256 checksum |
---|---|---|---|
Windows | commandlinetools-win-11076708_latest.zip | 153.6 MB | 4d6931209eebb1bfb7c7e8b240a6a3cb3ab24479ea294f3539429574b1eec862 |
Mac | commandlinetools-mac-11076708_latest.zip | 153.6 MB | 7bc5c72ba0275c80a8f19684fb92793b83a6b5c94d4d179fc5988930282d7e64 |
Linux | commandlinetools-linux-11076708_latest.zip | 153.6 MB | 2d2d50857e4eb553af5a6dc3ad507a17adf43d115264b1afc116f95c92e5e258 |
Command-line tools are included in Android Studio. If you do not need Android Studio, you can download the basic Android command-line tools above. You can use the included sdkmanager
to download other SDK packages.